TLDR; It’s easy to pre-populate the email field in a form using a few lines of Javascript. This method should work with any ESP that allows you to embed a subscriber email address as a URL parameter in a link.
By passing the email address in a URL, you can pre-populate the email field (even hide it altogether) and make surveys or feedback forms completely frictionless for existing subscribers.
You can use this to tag & segment existing subscribers extremely effectively, a feature usually reserved for pricey paid services like rightmessage.
What we’re building (demo above)
Above, you’ll find an example form that can pre-populate the email field from the query parameter in the URL.
Try it Yourself: Click the button to reload the page with a query string. You’ll note the form will be filled with the email shown in the URL. You can change the email address is in the URL, hit enter (to load the new URL) and note the form is filled with whatever email address to entered.
Why prepopulate a form?
I’ve been using Convertkit to grow my email list and engage my audience. One of my favorite Convertkit features is its robust automation system and subscriber tagging.
And I wanted to leverage this automation to let subscribers self-direct their content journey. They can fill out a short form or quiz and I’ll direct them to the customized email sequences. It’s a win-win. Except for one problem:
Convertkit (and many other ESP’s) don’t have a built-in way to recognize existing subscribers when they fill out a form. This causes two problems:
- Duplicate Subscribers: They may use a different email to complete the 2nd form, defeating the purpose and bloating your list.
- Abandonment: Seeing an empty email field, they may abandon the form altogether.
And I’m not alone in wanting this. I found several threads in the Convertkit forum asking for a solution to this problem.
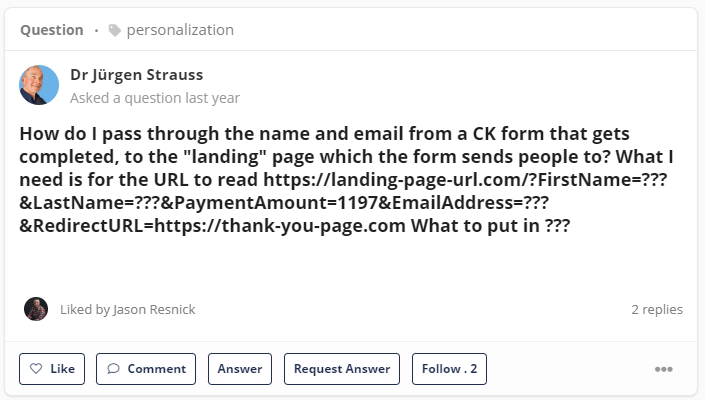
Why I want to do it
Surveying your list is incredibly valuable. Not only can you get an amazing insights about your audience, it’s also a fantastic segmentation opportunity.
For example, with just a few questions, I can find exactly where each subscriber is in their journey and meet them with the right content at the right moment.
Example Questions:
- How long have you been building websites?
- Total Beginner
- 1 year or less
- 2 – 5 years
- More than 5 years
- Who do you want to build websites for?
- Myself
- Clients
- What type of website(s) are you building?
- Blog / Affiliate
- E-commerce
- Business
- Personal
It’s easy to imagine how different the needs of each person will be, based on their answers. And with these insights I can help them achieve their goals faster and more effectively.
Existing Solutions
The only pre-built solution I’ve found (that integrates with Converkit anyway) is RightMessage.
RightMessage is a full-featured personalization platform that is amazingly powerful. Pat Flynn of Smart Passive Income uses RightMessage for this exact purpose, to segment his list. But he also uses it to personalize content on his website, gather user feedback, and more.
And while I think the product is fantastic, it’s also overkill. And pretty darn expensive if I’m being honest.
Typeform is also capable of this (on paid plans) if you use custom fields to grab the URL parameter. It would definitely work for me, but it costs nearly as much as my email list for what is in all honesty a pretty simple product.
My Solution: Javascript Snippet
I’m an amateur developer at best but this script was easy enough to put together.
Here’s what it does:
- Check if the page is loaded
- Pull the query string from the URL
- Find the ‘Subscriber’ parameter and store the value (email address) in a variable
- Fill the form’s email field with that email address
Easy Peezy!
The Code
function fillFormEmail(){
const input = document.getElementById('id'); // replace 'id' with id of email field
const queryString = window.location.search;
const urlParams = new URLSearchParams(queryString); //parse the query string
const email = urlParams.get('subscriber'); // Store the parsed email address in variable
input.value = email; // fill the form with the email address
}
if (document.readyState === 'loading') { // Loading hasn't finished yet
document.addEventListener('DOMContentLoaded', fillFormEmail)
}
else { // `DOMContentLoaded` has already fired
fillFormEmail();
}
Code language: JavaScript (javascript)
Usage Instructions
There are two things you have to do to make this code work for you:
- Add the id of your email form input in the line
document.getElementB
yId(‘id’); - Construct your link URL property (to pass the email)
Get the ID of your form field
- Using Chrome or Firefox, you can right-click the email field and choose ‘inspect’.
- Then find the the element <input type=”email” id=”some-id”> and note the text in the id attribute.
- Edit the javascript and replace ‘id’ with the id of your form (as shown below).
Pass the subscriber email as a URL Parameter
The exact instructions will vary depending on which email provider you use, but here’s the basic URL format to use:
https://yoursite.com/[email protected]
So all you have to do is add that last part, starting with the question mark.
Instructions for Convertkit
When you link to the form in your email, simply add this text string to the end of your url:
?subscriber={{ subscriber.email_address }}
That’s it. That shortcode will programmatically be replaced with the email address for each subscriber when your newsletter goes out.
You’re a lifesaver. I spent hours trying to figure this out. Don’t think I would’ve gotten there without finding this article. Thanks for putting it out there.
You’re welcome! I was having the same issues as you (there’s no easy solution available) so I figured out how to build it. In the future I’d like to build a complete email preferences page that lets subscribers opt in or out of specific sequences.
I normally pre fill forms this way with PHP a different way. I needed a javascript solution and this is just what I needed. Thank you for taking the time to post this!
I feel like I’ve searched for this before countless times and always end up reverting back to the php way I know. This is a very easy and straightforward solution that works, very happy with this
Awesome, glad to hear it was helpful! If it would be valuable for your prospects/clients please consider linking to or sharing this tutorial.
Hello Matthew! Thanks for sharing this. I’m not too code savvy but where do I add this javsacript code if I am to embed the form on my website?
You can just use inline JavaScript as I do in this article. Use an HTML block and paste the javascript code in between
tags.
Hi Matthew,
this looks like a great solution for GetResponse too. But what does the complete Code with tags look like?
I tried to add them myself, but the code is not working.
Thanks for any suggestions here.
I haven’t used GetResponse so you’d have to consult their documentation for the correct way to include the subscriber email address in your link URL.
When i am adding ?subscriber={{ subscriber.email_address }} to the end of my URL that is being clicked in my email, its not returning with the subscriber email but instead just including exactly that written in the string.
its coming back: subscriber=%7B%7B%20subscriber.email_address%20%7D%7D
If your email provider is encoding URLs, you can use the decodeURI() javascript function to convert the URL into the correct format. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/decodeURI